Table Of Content

Now let's explore the ways to implement the Factory design pattern together. From the class diagram, coders can easily see that the implementation for Factory is extremely straightforward. AccountFactory takes an AccountType as input and returns a specific BankAccount as output. The pattern allows you to produce different types and representations of an object using the same construction code. From Lehman’s point of view, we can say that a factory is a place where products are created. In order words, we can say that it is a centralized place for creating products.
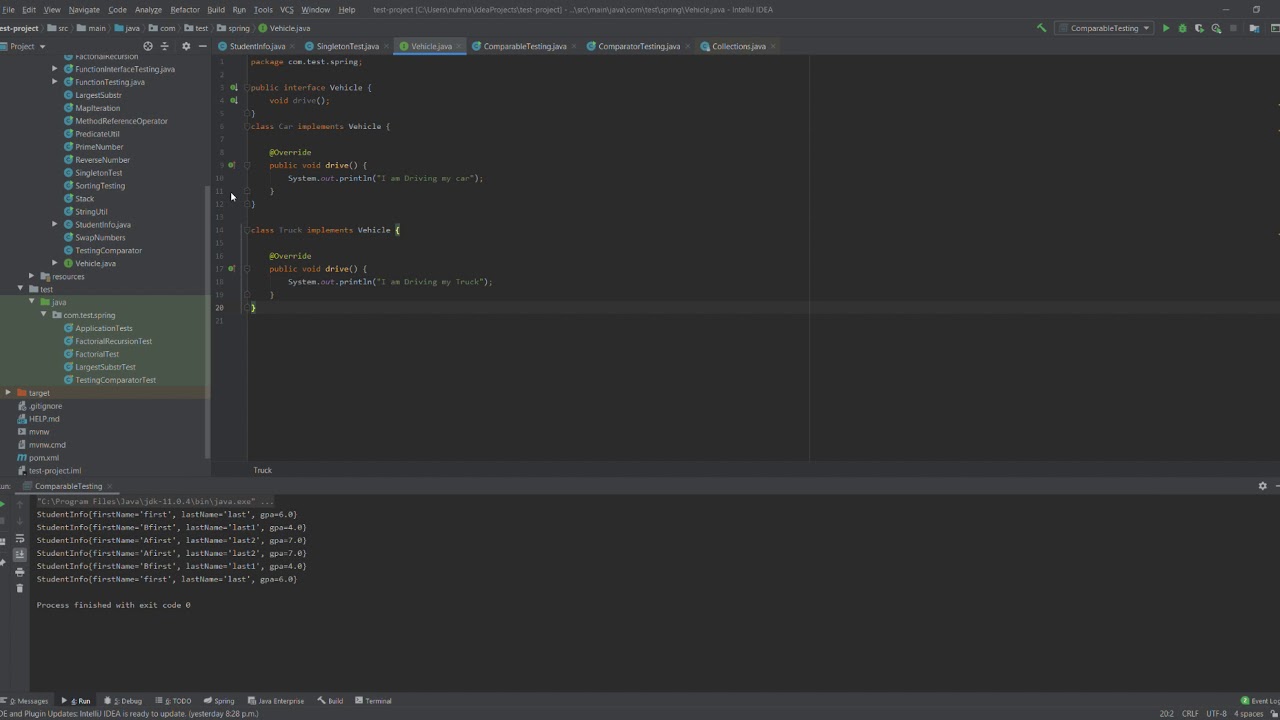
Types of Design Patterns in Java
The prototype design pattern mandates that the Object which you are copying should provide the copying feature. However, whether to use the shallow or deep copy of the object properties depends on the requirements and is a design decision. Creational design patterns provide solutions to instantiate an Object in the best possible way for specific situations. Java design patterns are divided into three categories - creational, structural, and behavioral design patterns.
6 State Method
This pattern is also used to provide different kinds of iterators based on our requirements. The iterator pattern hides the actual implementation of traversal through the Collection and client programs use iterator methods. Behavioral design patterns are a subsetof design patterns in software development that deal with the communication and interaction between objects and classes. They focus on how objects and classes collaborate and communicate to accomplish tasks and responsibilities. Singleton Method is a creational design pattern, it provide a class has only one instance, and that instance provides a global point of access to it.
Design Patterns in Java
It uses the accountType to create the corresponding type of BankAccount. A factory (i.e. a class) will create and deliver products (i.e. objects) based on the incoming parameters. The memento design pattern is used when we want to save the state of an object so that we can restore it later on. This pattern is used to implement this in such a way that the saved state data of the object is not accessible outside of the Object, this protects the integrity of saved state data. We know that we can have multiple catch blocks in a try-catch block code.
Template Method Pattern
The Factory design pattern is one of the creational patterns and we can find its uses in almost all the libraries in JDK, Spring. We will understand what this pattern is about using a Java application. It is a creational design pattern that talks about the creation of an object. The factory design pattern says to define an interface ( A java interface or an abstract class) for creating the object and let the subclasses decide which class to instantiate.
8 Template Method
You are developing a software system for an e-commerce platform that deals with various types of products. Each product category (e.g., electronics, clothing, books) requires specific handling during creation. However, you want to decouple the client code from the concrete product creation logic to enhance flexibility and maintainability. Additionally, you want to allow for easy extension by adding new product types in the future without modifying existing code. Structural design patterns are a subset of design patterns in software development that focus on the composition of classes or objects to form larger, more complex structures.
Step 1
Adding Ships into the app would require making changes to the entire codebase. Moreover, if later you decide to add another type of transportation to the app, you will probably need to make all of these changes again. Design patterns are solutions to general problems that software developers faced during robust scalable software development. To master the Factory Design Pattern in Java, it's beneficial to collaborate with seasoned professionals.
Use Cases of the Factory Method Design Pattern in Java
It's a misconception that design patterns are holy solutions to all problems. Design patterns are techniques to help mitigate some common problems, invented by people who have solved these problems numerous times. To solve this problem, we can create a Factory of spaceships and leave the specifics (which engine or dish is used) to the subclasses to define.
You should favor composition over inheritance in Java. Here's why. - Oracle
You should favor composition over inheritance in Java. Here's why..
Posted: Thu, 14 Jul 2022 07:00:00 GMT [source]
When the factory method comes into play, you don’t need to rewrite the logic of the Dialog class for each operating system. If we declare a factory method that produces buttons inside the base Dialog class, we can later create a subclass that returns Windows-styled buttons from the factory method. The subclass then inherits most of the code from the base class, but, thanks to the factory method, can render Windows-looking buttons on the screen.
The abstract factory pattern is similar to the factory pattern and is a factory of factories. Creational design patterns are a subset of design patterns in software development. They deal with the process of object creation, trying to make it more flexible and efficient. It makes the system independent and how its objects are created, composed, and represented. The factory design pattern offers a solution for efficient object creation in software development.
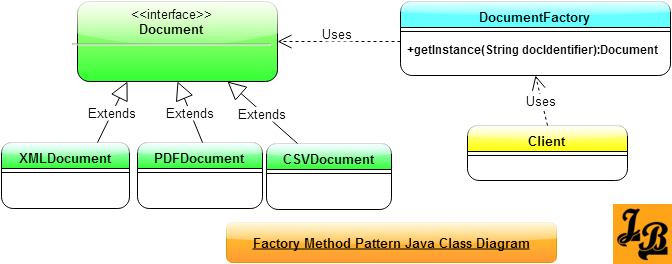
The Factory Pattern is a powerful design pattern that simplifies object creation, enhances code organization, and promotes maintainability. By abstracting the creation process and delegating it to subclasses or specialized factories, it enables flexible, extensible, and clean code. The factory class is the main component of the factory design pattern. This class return the object of required class based on the input parameter. If you observe, the main purpose of our factory class is to return the object at run time.
Please read our previous article where we give a brief introduction to the Creational Design Pattern. The Factory Design Pattern is one of the most frequently used design patterns in real-time applications. The Factory Design Pattern falls under the Creational Design Patterns Category. Strategy pattern is used when we have multiple algorithms for a specific task, and the client decides the actual implementation be used at runtime. We define multiple algorithms and let client applications pass the algorithm to be used as a parameter.
The Factory Design Pattern in C++ involves using a base class or interface with a virtual method for creating objects. Derived classes can override this method to create different object types based on client requirements. This pattern promotes flexibility and extensibility by letting subclasses decide which class to instantiate. It simplifies object creation without needing to know specific types, making code modular and easier to maintain. Additionally, it enables the creation of complex objects that may be challenging to create manually. At its core, the Factory Pattern is a creational design pattern that abstracts the process of object creation.
If you change the original code, then the method itself looses the point because it needs to be rewritten every time someone wants to make a small change to the ship. Since the instantiations are hard-coded, you could either create a duplicate of your original method or change its code. Specifically, the Factory Method and Abstract Factory are very common in Java software development. The Factory Method Pattern is one of several Creational Design Patterns we often use in Java. Their purpose is to make the process of creating objects simpler, more modular, and more scalable. I hope, I have included enough information in this Java factory pattern example to make this post informative.
However, this will lead to the create(AccountType type) method becoming increasingly bloated. The first strength of the Factory pattern that we can see is that it centralizes the creation of objects in one place. The challenge is to find a suitable solution to create these types of Banking Accounts.
That method will create and return different types of objects based on the input parameter, it received. In this article, we’ve explored the Factory Pattern in the context of a food ordering system. Keep in mind that this is just one of many applications for this pattern. The implementation of the bridge design pattern follows the notion of preferring composition over inheritance. The builder pattern was introduced to solve some of the problems with factory and abstract Factory design patterns when the object contains a lot of attributes. Flyweight Method is a structural design pattern, it is used when we need to create a lot of objects of a class.
No comments:
Post a Comment